This series of articles covers basic low-level concepts in computers, with a specific focus on exploring binaries and reverse engineering. Some points may seem confusing at first, but remember: everything follows a definition. Don’t get stuck in an endless loop of “why!?!” — just understand each concept and its purpose. You will use these concepts in reverse engineering, binary exploitation, and even malware development in the future.
What is a CPU? Link to heading
The Central Processing Unit (CPU) is the “brain” of a computer, responsible for processing information and managing data stored in memory. It performs essential functions such as processing, storage, and input/output operations, making it crucial for the functioning of computer systems, including operating systems, mobile devices, and embedded systems.
A computer, in a simplified way, can be seen as a set of instructions stored in memory that are processed by the CPU. The basic architecture of a computer consists of three main components: the CPU, memory, and input/output devices, all connected through a bus (BUS) (see the image above).
The foundation of this architecture was proposed by John Von Neumann, whose concepts still influence the design of modern processors. However, contemporary CPUs often do not strictly follow Von Neumann’s original architecture, although many of its principles remain fundamental. Additionally, CPUs may adopt different internal management models, such as CISC (Complex Instruction Set Computing) and RISC (Reduced Instruction Set Computing), which will be explained later.
Components of a Computer Link to heading
CPU internal components Link to heading
Registers Link to heading
Registers are internal storage areas of the CPU, designed to process data quickly. Unlike RAM, where data stays for a longer period, data in registers is temporary and used in high-speed operations. Before being processed, all data must be stored in a register. (This concept will be better understood when we cover Assembly language).
Arithmetic and Logic Unit (ALU) Link to heading
The ALU (Arithmetic and Logic Unit) is responsible for performing arithmetic calculations (such as addition and subtraction) and logical operations (such as AND, OR, NOT) that have been decoded by the Control Unit (CU).
Some of the most common processes executed by the ALU include:
- Addition
- Subtraction
- Division
- Multiplication
- Selection / Branching
- Repetition / Looping
Control Unit (CU) Link to heading
The Control Unit (CU) is the part of the CPU responsible for coordinating and managing the system’s operations. It controls input and output (I/O) operations, ALU (Arithmetic and Logic Unit) operations, memory access, and interaction with peripheral devices. The CU interprets the program instructions and ensures that each component of the CPU performs its tasks in an orderly and efficient manner, guaranteeing the correct functioning of the system.
Cache Link to heading
The cache is a high-speed memory located close to the CPU that helps accelerate operations.
Types of Cache: Link to heading
Types of Cache: Link to heading
- L1 Cache:
- Integrated into the processor or close to it.
- Smaller capacity but faster.
- Stores frequently accessed data (e.g., registers).
- Size: 2 KB to 64 KB.
- L2 Cache:
- Can be integrated into the processor or located close to it.
- Larger capacity than L1.
- Acts as an intermediary between L1 and the rest of the memory.
- Size: 256 KB to 512 KB.
- L3 Cache:
- Located outside the processor.
- Increases the performance of L1 and L2.
- Larger capacity than L1 and L2.
- Size: 1 MB to 8 MB.
In summary, each cache level stores data hierarchically, optimizing CPU performance.
Memory Management Unit (MMU) Link to heading
The Memory Management Unit (MMU) is responsible for assisting the operating system in managing memory resources efficiently. It controls the allocation and release of memory, as well as translating virtual addresses to physical addresses, allowing the operating system to access and manipulate data securely and optimally. The MMU is also essential for managing virtual memory, ensuring that processes are isolated and memory is used effectively.
Firmware Link to heading
Firmware is a type of fixed, specialized program embedded directly into a device’s hardware, responsible for the initialization and basic configuration of the system. It provides the necessary instructions for the hardware to function correctly and starts the process of loading the operating system. Firmware is essential for the initial operation of devices such as computers, printers, and routers.
External CPU Link to heading
Main Memory (RAM) Link to heading
RAM (Random Access Memory) is a volatile memory that stores temporary data while the system is running. It organizes this data in sequences of bytes, with each byte having a unique address used for quick access. The amount of available RAM determines the physical memory of a device or computer. Currently, RAM capacities can range from gigabytes (GB) and are used to store temporary data, such as the operating system and specific areas for buffers and input/output (I/O) devices.
The image below illustrates how a 16 GB RAM operates in a modern system:
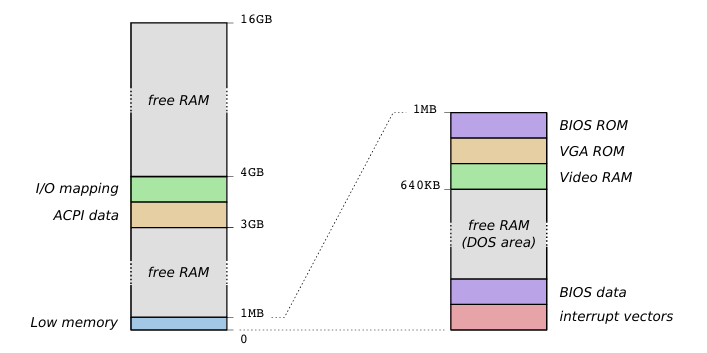
- Free: Refers to the memory spaces available for the operating system and for managing running processes.
The other memory spaces are used for hardware management, input/output (I/O) operations, and the operating system.
Currently, physical memory is not accessed directly by processes. Instead, it is mapped through address spaces and virtual memory, allowing the operating system to manage and allocate memory efficiently, isolating processes and maximizing the use of available resources.
External Storage (e.g., Hard Drives, USB Drives, etc.) Link to heading
External storage devices are non-volatile memories, meaning they retain data even when the system is turned off. They offer much larger storage capacities compared to RAM, but, in contrast, tend to be slower in terms of access speed. These devices are used to store large volumes of data permanently, such as files, programs, and operating systems.
Ports Link to heading
Ports are the physical or logical access points that allow peripherals to be connected to the computer. Devices such as printers, keyboards, mice, and other accessories are connected through these ports. Drivers are specific programs that manage communication between the operating system and the peripherals, controlling how these devices are handled and interacting with the computer.
Bus Link to heading
The bus is a set of physical or logical pathways in a system that allows data transfer between the different components of the computer. It facilitates communication between the CPU, memory, and peripherals.
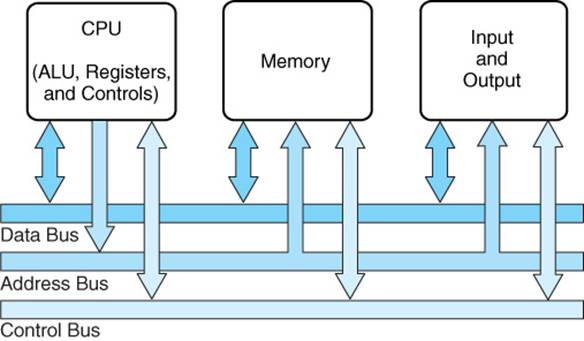
The three main types of lines in a bus are:
- Control line: Responsible for managing control and synchronization operations between components.
- Address line: Carries memory or device addresses to specify where data should be read or written.
- Data line: Transfers the actual data between components.
How is a program executed? Link to heading
When we open a program, it is loaded into the computer’s memory. A program is simply a set of instructions in machine code that the CPU must execute.
In a 32-bit CPU (like the x86 architecture), it reads small blocks of data called doublewords (which are 4 bytes or 32 bits). When it encounters an address in memory, the CPU takes that data block and temporarily stores it in one of its registers, which are like quick drawers. Then, the CPU reads the content of this data and executes the instruction contained within.
After executing each instruction, the CPU fetches the next instruction from memory.
The CPU has a register called the Instruction Pointer (IP), or EIP (Extended Instruction Pointer) / RIP (Register Instruction Pointer), which points to the address of the next instruction to be executed.
This register can be manipulated, altering the flow of the program. This is exploited by malwares to modify the system’s behavior.
Risc vs. Cisc Link to heading
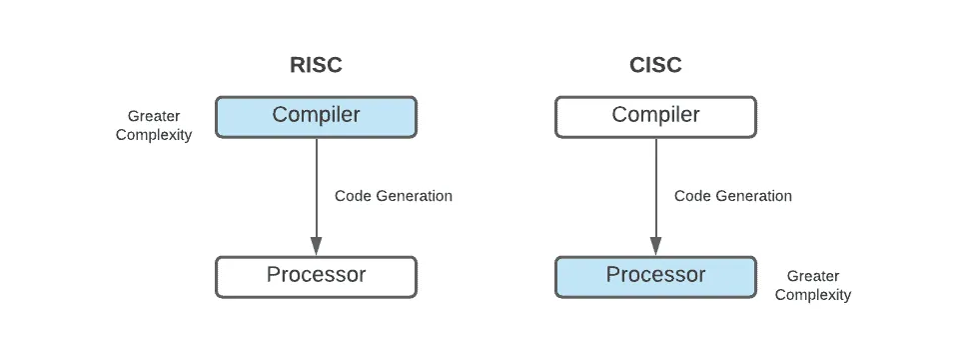
CISC Link to heading
CISC (Complex Instruction Set Computer) is a processor architecture that prioritizes powerful instructions with multiple steps or functionalities. It was designed to minimize the number of instructions the programmer needs to write by offering commands with various functionalities. This architecture is very common in personal computers and servers, being used by companies like Intel and AMD.
Key Features of CISC: Link to heading
- Complex and multi-step instructions: Many CISC instructions perform multiple operations at once, reducing the number of instructions needed, but it also decreases the operation speed.
- Variable instruction lengths: Instructions can be of different sizes.
- Direct memory access: CISC instructions can access memory directly, which simplifies programming, but increases data access time.
- Advanced addressing modes: CISC has many forms of addressing, resulting in flexibility to manage data structures…
Example of a CISC instruction Link to heading
In x86 assembly, a single instruction can combine:
- Data loading
- Arithmetic (calculations)
See:
mov eax, [ebx]
This single line moves (loads) the value from the memory address contained in ebx
to the eax
register.
Components: Link to heading
- mov: This is the move register, used to copy data from one place to another. In x86 assembly,
mov
is used to load data from memory, or from another register, into a specific register. - eax: This is the destination register, meaning the place where the data will be copied.
- [ebx]: This indicates the source operand. The brackets around
ebx
indicate that the value being accessed is not directly in theebx
register, but at the memory address stored in theebx
register. In other words,ebx
contains a memory address, and the value at that address will be moved to theeax
register.
Advantages of CISC Link to heading
- Backward compatibility: CISC architectures like x86 maintain compatibility with older generations. This allowed older software to run on current systems.
- Versatile performance: With the flexibility that CISC offers, it is ideal for general-purpose computing.
CISC has its advantages for computers where resources are more abundant. However, it consumes more power and generates heat, which is unsuitable for mobile devices, where efficiency is key. Nowadays, CISC uses internal structures similar to RISC to improve efficiency.
RISC Link to heading
RISC (Reduced Instruction Set Computer) is a processor architecture/design focused on simplicity and speed. In RISC architectures like ARM and RISC-V, instructions are designed to be executed in a single clock cycle, which increases execution speed and reduces power consumption. This makes RISC ideal for mobile devices, embedded systems, and efficient computing.
Key Features of RISC: Link to heading
- Simple single-cycle instructions: RISC instructions are simple, allowing for fast execution.
- Fixed-length instructions: Instructions have a fixed length, reducing decoding complexity, which results in increased execution speed.
- Load/store architecture: RISC separates memory access and computation. It requires that data be loaded into registers first, and then operated on. This means that before performing any arithmetic or logical operation on data, the data must first be loaded (load) into a register. After manipulation, the result can be stored (store) back into memory.
- Limited addressing modes: RISC has fewer addressing instructions, which reduces complexity. However, this requires additional instructions for more complex operations.
Example of a RISC instruction sequence Link to heading
In ARM assembly, many instructions separate the loading and processing of data.
Load Data from Memory to Registers Link to heading
ldr r0, [r1] ; Carrega o valor do endereço de memória armazenado em r1 para o registrador r0
add r0, r0, #1 ; Adiciona 1 ao valor de r0
str r0, [r1] ; Armazena o resultado da operação no endereço de memória indicado por r1
Here, we load the value from memory into a register, perform the operation, and then store the result back into memory. It’s simple and efficient.
Advantages of RISC Link to heading
-
Energy efficiency: Due to its simple instructions, the RISC architecture consumes less power, making it ideal for mobile devices and IoT (Internet of Things).
-
Scalability and flexibility: RISC architectures, like ARM, can be used in a wide range of devices, from simple mobile devices to large data centers.
x86 Architecture Link to heading
The x86 architecture originated with the 8086 microprocessor, developed by Intel. Due to its success, the company expanded the line, releasing new models such as 80186, 80286, 80386, and so on.

Initially, the naming followed the 80x86 standard, where the “x” represented a variable number. Over time, for simplification, the name was shortened to x86, becoming the widely used term to this day. With the evolution of technology, the x86 architecture received various improvements and extensions, always preserving compatibility with previous generations. This principle allowed older software to continue running on more modern processors, ensuring a smooth transition between different hardware generations.
One of the most significant changes came with AMD, which developed the x86-64 architecture, introducing support for 64-bit. This innovation allowed for greater processing power and support for more RAM, marking a significant advance in high-performance computing.
Although Intel initially invested in its own 64-bit architecture, called IA-64 (used in the Itanium line), it eventually adopted AMD’s x86-64 standard. This ensured that processors from both companies were compatible and followed the same instruction set, promoting a joint evolution of the architecture.
Today, Intel and AMD continue to advance the x86-64 architecture, incorporating new technologies, improving energy efficiency, and enhancing performance, while always maintaining compatibility.
Official Name | Alternative Name | Bit | Instruction Set | Design |
---|---|---|---|---|
8086 | IA-16 | 16 | x86 | CISC |
Official Name | Alternative Name | Bit | Instruction Set | Design |
---|---|---|---|---|
IA-32 | i386 | 32 | x86 | CISC |
Official Name | Alternative Name | Bit | Instruction Set | Design |
---|---|---|---|---|
x86-64 | i686 | 64 | x86-64 (AMD64) | CISC |